Introduction
BTPayments is the world’s leading cryptocurrency payment service provider, offering global payment solutions for over 100 industries such as live streaming, dating, and VPN. With its robust blockchain infrastructure, BTPayments supports over 170 wallets, covering more than 50 cryptocurrencies including bitcoin, ethereum, USDT, and more.
Integration steps for BTPayments SDK
Step 1: Account registration
Contact your account manager or register through the merchant’s backend at BTPayments merchant portal to obtain your BTPayments account. Log in to the portal to access the relevant secret key.
Step 2: SDK download and configuration
Download the corresponding SDK from our SDK download list and extract the files. Insert the secret key obtained from the merchant portal into the corresponding SDK configuration file. If your preferred development language is not listed in our SDKs, please refer to our development documentation.
Step 3: SDK installation and deployment
Install and deploy the downloaded SDK in your development environment. Once installed, you can make API calls to request payments and query related interfaces. For code samples, please refer to our payment interface documentation.
Step 4: Webhook configuration
Set up the webhook to receive asynchronous callbacks for your transactions. Webhooks allow you to process transaction updates in real-time. Configure the webhook URL in the BTPayments Merchant Portal to receive notifications. For more information, please see our documentation on webhooks.
Step 5: Transaction handling
Process relevant transaction information promptly based on the webhook notifications received. Update the transaction status within your system accordingly.
Please note that the provided steps serve as a general guideline for integrating the BTPayments SDK. For more detailed instructions and a comprehensive API reference, refer to our complete documentation available in the BTPayments Merchant Portal.
API Guidelines
1.System flow
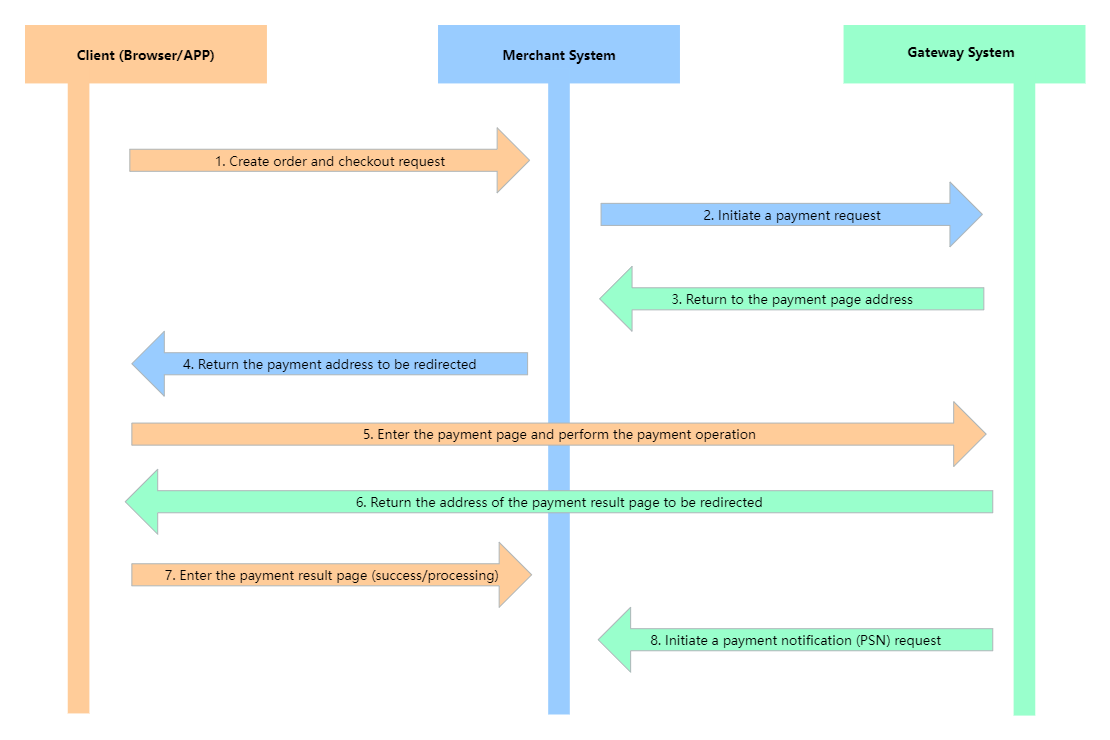
The main payment process follows these steps:
- The end customer (payer) creates an order in the merchant system.
- The merchant system (backend server) initiates a payment request to the BTPayments gateway.
- The BTPayments gateway returns a response containing the payment page address.
- The terminal browser redirects to the payment page.
- After the customer completes the payment, the terminal browser redirects to the merchant system’s success page.
- The BTPayments gateway sends a payment status notification (PSN) request to the merchant system.
2.Protocol
The communication protocol between the API and client users uses the HTTPS protocol to ensure secure transmission of interactive data.
3.Domain name
Production Environment – https://pay.btpayments.io
4.HTTP request method
All requests to the CoinPal gateway must be made using the POST method.
5.Signature algorithm
All data signatures are encrypted using the SHA256 algorithm.
6.Character encoding
All data must be encoded in UTF-8 format to avoid unexpected errors.
7.Response data
The data returned by the CoinPal gateway is in JSON format.
Quick Start
Step 1: The client generates a payment order using the following code and quickly redirects to the BTPayments cash register.
BTPayments cash register-DOME (production environment)
Please modify the parameter values in the request as needed, such as requestId, merchantNo, accessToken, orderNo, and so on.
Step 2: Process relevant transaction information in a timely manner based on the webhook address to update the transaction status in your system (View webhook configuration)
- To ensure data security, it is necessary to perform signature verification (Authentication and Security).
- Additionally, you can query transaction details for data security (Get payment status).
Create Transaction
The merchant system initiates a payment request to the CoinPal gateway through HTTP POST:
Request parameters
The request parameters contain all the information related to the payment. A basic payment request should include the following required parameters:
Parameter | Description | Required | Length | Example |
---|---|---|---|---|
version | Interface version number. | Yes | 10 | 2 |
requestId | Unique serial number for each request. | Yes | 256 | requestId123 |
merchantNo | Merchant number. | Yes | 64 | 100000000 |
orderNo | Merchant order number. | Yes | 256 | Test-001
|
orderCurrencyType | Currency type: “fiat” (legal currency) or “crypto” (digital currency). | Yes | 16 | fiat |
orderCurrency | Order currency. (view order currency list) | Yes | 10 | USD |
orderAmount | Order amount. | Yes | 16 | 10 |
notifyURL | Merchant’s asynchronous notification URL. | Yes | 256 | https://www.btpayments.io/notification |
redirectURL | Callback address of the front page after successful/expired payment by the user. | Yes | 256 | https://www.btpayments.io/redirect |
cancelURL | Callback address of the front page when the user cancels the payment. Default is the redirectURL if not provided. | No | 256 | https://www.btpayments.io/cancel |
successUrl | Redirect URL for successful payment. If empty, redirectURL will be used by default. | No | 256 | https://www.btpayments.io/success |
sign | Digital signature of the current request. Required when accessToken is empty. view digital signature | No | 64 | 8a8d17fb8f50db848c8aa2f850e83688c9ceb611cea761f0c64b998c5bbd7e4e |
accessToken | accessToken of the current request. Required when sign is empty. view accessToken | No | 64 | 550e07b3 |
nextStep | Requested mode. When set to “form”, it directly jumps to the payment page. | No | 20 | form |
payerIP | Payer’s device IP. | No | 256 | 192.168.1.100
|
merchantName | Merchant name displayed on the cash register page. | No | 256 | BTPayments |
payerEmail | User’s email. | No | 256 | customer@example.com |
orderDescription | Order description displayed on the cash register page. | No | 256 | Order description
|
resultNotifyUser | Whether BTPayments will send the final confirmation result of the transaction to the user. | No | 10 | True |
unpaidAutoRefund | Whether CoinPal should automatically refund in case of underpayment or overpayment by the user. | No | 10 | True |
remark | Extended field that can be defined by merchants. Will be returned as it is after the payment is successful. | No | 500 | remark |
cryptoCurrency | Specified currency | No | 10 | KAS |
network | Designated public chain (KAS,BITCOIN,ERC20,TRC20) | No | 50 | KAS |
walletId | Specify wallet ID (view wallet list) | No | 10 | 645 |
syncMethod | Synchronous notification method (get, post) | 否 | 10 | get |
Code example
POST – https://pay.btpayments.io/gateway/pay/checkout
PHP Request example:
$url = "https://pay.coinpal.io/gateway/pay/checkout";
$data['version'] = '2';
$data['requestId'] = 'RE1686722509';
$data['merchantNo'] = '100000000';
$data['orderNo'] = 'OR1686722509';
$data['orderCurrencyType'] = 'fiat';
$data['orderCurrency'] = 'EUR';
$data['orderAmount'] = '12';
$data['notifyURL'] = 'https://www.coinpal.io';
$data['redirectURL'] = 'https://www.coinpal.io';
$data['accessToken'] = '550e07b3';
function curl_request($url,$pdata){
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $pdata);
curl_setopt($ch, CURLOPT_TIMEOUT,60);
$result = curl_exec($ch);
if($result === false){
return curl_error($ch);
}
curl_close($ch);
return $result;
}
$order_data = curl_request($url, $data);
echo $order_data;
Return example:
{
"version": "2",
"requestId": "RE1686722509",
"merchantNo": "100000000",
"orderNo": "OR1686722509",
"reference": "CWS1U15BQPGXZ9WJ",
"orderCurrency": "EUR",
"orderAmount": "12",
"nextStep": "redirect",
"nextStepContent": "https://pay.coinpal.io/cashier/wallet/web/30af1acbd1742eba8e987c882430fd05",
"status": "created",
"respCode": 200,
"respMessage": "success",
"remark": null
}
Java Request example:
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
String url = "https://pay.coinpal.io/gateway/pay/checkout";
Map data = new HashMap<>();
data.put("version", "2");
data.put("requestId", "RE1686722509");
data.put("merchantNo", "100000000");
data.put("orderNo", "OR1686722509");
data.put("orderCurrencyType", "fiat");
data.put("orderCurrency", "EUR");
data.put("orderAmount", "12");
data.put("notifyURL", "https://www.coinpal.io");
data.put("redirectURL", "https://www.coinpal.io");
data.put("accessToken", "550e07b3");
try {
String orderData = sendPostRequest(url, data);
System.out.println(orderData);
} catch (Exception e) {
e.printStackTrace();
}
}
public static String sendPostRequest(String url, Map data) throws Exception {
URL obj = new URL(url);
HttpURLConnection connection = (HttpURLConnection) obj.openConnection();
connection.setRequestMethod("POST");
connection.setDoOutput(true);
StringBuilder postData = new StringBuilder();
for (Map.Entry param : data.entrySet()) {
if (postData.length() != 0) postData.append('&');
postData.append(param.getKey());
postData.append('=');
postData.append(param.getValue());
}
byte[] postDataBytes = postData.toString().getBytes("UTF-8");
connection.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
connection.setRequestProperty("Content-Length", String.valueOf(postDataBytes.length));
try (OutputStream os = connection.getOutputStream()) {
os.write(postDataBytes);
}
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
StringBuilder response = new StringBuilder();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
return response.toString();
}
}
Return example:
{
"version": "2",
"requestId": "RE1686722509",
"merchantNo": "100000000",
"orderNo": "OR1686722509",
"reference": "CWS1U15BQPGXZ9WJ",
"orderCurrency": "EUR",
"orderAmount": "12",
"nextStep": "redirect",
"nextStepContent": "https://pay.coinpal.io/cashier/wallet/web/30af1acbd1742eba8e987c882430fd05",
"status": "created",
"respCode": 200,
"respMessage": "success",
"remark": null
}
Go Request example:
package main
import (
"bytes"
"fmt"
"io/ioutil"
"net/http"
)
func main() {
url := "https://pay.coinpal.io/gateway/pay/checkout"
data := map[string]string{
"version": "2",
"requestId": "RE1686722509",
"merchantNo": "100000000",
"orderNo": "OR1686722509",
"orderCurrencyType": "fiat",
"orderCurrency": "EUR",
"orderAmount": "12",
"notifyURL": "https://www.coinpal.io",
"redirectURL": "https://www.coinpal.io",
"accessToken": "550e07b3",
}
orderData, err := sendPostRequest(url, data)
if err != nil {
fmt.Println(err)
return
}
fmt.Println(orderData)
}
func sendPostRequest(url string, data map[string]string) (string, error) {
postData := []byte{}
for key, value := range data {
postData = append(postData, []byte(key+"="+value+"&")...)
}
postData = postData[:len(postData)-1] // Remove the trailing '&'
resp, err := http.Post(url, "application/x-www-form-urlencoded", bytes.NewReader(postData))
if err != nil {
return "", err
}
defer resp.Body.Close()
responseData, err := ioutil.ReadAll(resp.Body)
if err != nil {
return "", err
}
return string(responseData), nil
}
Return example:
{
"version": "2",
"requestId": "RE1686722509",
"merchantNo": "100000000",
"orderNo": "OR1686722509",
"reference": "CWS1U15BQPGXZ9WJ",
"orderCurrency": "EUR",
"orderAmount": "12",
"nextStep": "redirect",
"nextStepContent": "https://pay.coinpal.io/cashier/wallet/web/30af1acbd1742eba8e987c882430fd05",
"status": "created",
"respCode": 200,
"respMessage": "success",
"remark": null
}
Python Request example:
import requests
url = "https://pay.coinpal.io/gateway/pay/checkout"
data = {
'version': '2',
'requestId': 'RE1686722509',
'merchantNo': '100000000',
'orderNo': 'OR1686722509',
'orderCurrencyType': 'fiat',
'orderCurrency': 'EUR',
'orderAmount': '12',
'notifyURL': 'https://www.coinpal.io',
'redirectURL': 'https://www.coinpal.io',
'accessToken': '550e07b3'
}
def send_post_request(url, data):
try:
response = requests.post(url, data=data)
response.raise_for_status()
return response.text
except requests.exceptions.RequestException as e:
return str(e)
order_data = send_post_request(url, data)
print(order_data)
Return example:
{
"version": "2",
"requestId": "RE1686722509",
"merchantNo": "100000000",
"orderNo": "OR1686722509",
"reference": "CWS1U15BQPGXZ9WJ",
"orderCurrency": "EUR",
"orderAmount": "12",
"nextStep": "redirect",
"nextStepContent": "https://pay.coinpal.io/cashier/wallet/web/30af1acbd1742eba8e987c882430fd05",
"status": "created",
"respCode": 200,
"respMessage": "success",
"remark": null
}
C# Request example:
using System;
using System.Collections.Generic;
using System.Net.Http;
using System.Threading.Tasks;
class Program
{
static async Task Main()
{
string url = "https://pay.coinpal.io/gateway/pay/checkout";
Dictionary data = new Dictionary
{
{ "version", "2" },
{ "requestId", "RE1686722509" },
{ "merchantNo", "100000000" },
{ "orderNo", "OR1686722509" },
{ "orderCurrencyType", "fiat" },
{ "orderCurrency", "EUR" },
{ "orderAmount", "12" },
{ "notifyURL", "https://www.coinpal.io" },
{ "redirectURL", "https://www.coinpal.io" },
{ "accessToken", "550e07b3" }
};
string orderData = await SendPostRequest(url, data);
Console.WriteLine(orderData);
}
static async Task SendPostRequest(string url, Dictionary data)
{
using (HttpClient httpClient = new HttpClient())
{
try
{
var content = new FormUrlEncodedContent(data);
var response = await httpClient.PostAsync(url, content);
response.EnsureSuccessStatusCode();
return await response.Content.ReadAsStringAsync();
}
catch (HttpRequestException e)
{
return e.Message;
}
}
}
}
Return example:
{
"version": "2",
"requestId": "RE1686722509",
"merchantNo": "100000000",
"orderNo": "OR1686722509",
"reference": "CWS1U15BQPGXZ9WJ",
"orderCurrency": "EUR",
"orderAmount": "12",
"nextStep": "redirect",
"nextStepContent": "https://pay.coinpal.io/cashier/wallet/web/30af1acbd1742eba8e987c882430fd05",
"status": "created",
"respCode": 200,
"respMessage": "success",
"remark": null
}
Transaction Query
BTPayments gateway provides a real-time transaction status query interface:
As cryptocurrencies payment is conducted through address transfers, we are unable to control user payment actions. to ensure merchants receive all payment notifications, for orders in their final states (such as Expired, Paid, Partial_Paid), merchants will receive asynchronous notification of each payment made by users. if you cannot manage such orders, you can deactivate these notifications in the CoinPal.io backend under My Account > Flexible Payment > Unresolved Notification.
Query parameters
The merchant system can query information such as the status of the specified transaction through this interface. A query request that meets the requirements will contain the following necessary parameters:
Request parameter name | Parameter description | Required | Maximum length | Parameter value example |
---|---|---|---|---|
reference | The reference field after the transaction is successfully created | Yes | 24 | CWSBV15AR219CQ1C |
The following are the parameter names of the response
Response parameter name | Parameter description | Maximum length | Parameter value example |
---|---|---|---|
version | The requested version number | 10 | 2 |
requestId | Request serial number | 256 | SN230529221238495453 |
merchantNo | Requested Merchant ID | 64 | 10290001 |
orderNo | Requested order number | 256 | GLD1685398357965285 |
reference | BTPayments gateway order number | 64 | CWSBV15AR219CQ1C |
orderCurrency | requested order currency | 10 | EUR |
orderAmount | requested order amount | 16 | 12 |
nextStep | next step | 64 | display_collection |
nextStepContent | Next Step Content | 64 | 3GJsmsMsRs4k5uGDUeRtfcWkwmovHfrtve |
status | order status (check order status) | 50 | paid |
respCode | The status code of the response | 10 | 200 |
respMessage | response message | 256 | success |
remark | Requested extension fields | 500 | remark |
Example of a successful query request
POST https://pay.btpayments.io/gateway/pay/query
reference=CWSBV15AR219CQ1C
Return normally
{
"version": "2",
"requestId": "SN230529221238495453",
"merchantNo": "18",
"orderNo": "GLD1685398357965285",
"reference": "CWSBV15AR219CQ1C",
"orderCurrency": "EUR",
"orderAmount": "66.54",
"nextStep": "display_collection",
"nextStepContent": "3GJsmsMsRs4k5uGDUeRtfcWkwmovHfrtve",
"status": "paid",
"respCode": 200,
"respMessage": "success",
"remark": ""
}
Webhook Configuration
When the status of each transaction is updated, such as payment success or failure, the BTPayments gateway system will initiate a notification request to the merchant system. the specific address of this request is provided by the merchant system in the notifyURL parameter of the payment request.
The notifyURL parameter should contain the address where the merchant system expects to receive the notification request. it is an asynchronous notification URL that BTPayments will use to send transaction-related data to the merchant system. the merchant system should be prepared to handle this notification and process the data accordingly.
Please ensure that the notifyURL parameter in the payment request is correctly set to the desired address where you want to receive the transaction status updates.
CoinPal gateway request merchant parameters
Request parameter name | Parameter description | Maximum length | Parameter value example |
---|---|---|---|
version | The requested version number | 10 | 2 |
requestId | Request serial number | 256 | SN230529221238495453 |
merchantNo | Requested Merchant ID | 64 | 10290001 |
orderNo | Requested order number | 256 | GLD1685398357965285 |
reference | CoinPal gateway order number | 64 | CWSBV15AR219CQ1C |
orderCurrency | requested order currency | 10 | EUR |
orderAmount | requested order amount | 16 | 12 |
paidOrderAmount | Order amount after successful payment | 16 | 12 |
selectedWallet | wallet id | 16 | 9999 |
dueCurrency | Due Currency | 10 | BTC |
dueAmount | Amount Due | 16 | 0.00047364 |
paidCurrency | Actual payment currency | 10 | BTC |
paidAmount | Actual payment amount | 16 | 0.00047364 |
paidUsdt | converted usdt amount (exchange rate fee will be deducted) | 16 | 12.8340 |
paidAddress | paidAddress | 256 | 3GJsmsMsRs4k5uGDUeRtfcWkwmovHfrtve |
confirmedTime | Timestamp of successful payment | 11 | 1685399666 |
status | order status (check order status) | 50 | paid |
remark | Requested extension fields | 500 | remark |
unresolvedLabel | exception label | 50 | overpaid |
sign | Digital signature of the current request (view digital signature) | 64 | 8a8d17fb8f50db848c8aa2f850e83688c9ceb611cea761f0c64b998c5bbd7e4e |
A parameter containing a digital signature will ensure the legitimacy of the current request (see digital signature for the specific algorithm). the merchant system can perform related business processing (such as updating the order status or preparing to ship, etc.) according to the status information in the request.
CoinPal gateway notification request example
POST https://www.merchant77.com/notify.php?order=ORDER1234567890
&version=2
&requestId=SN230529221238495453
&merchantNo=18
&orderNo=GLD1685398357965285
&reference=CWSBV15AR219CQ1C
&orderCurrency=EUR
&orderAmount=66.54
&paidOrderAmount=66.54000000
&selectedWallet=9999
&dueCurrency=BTC
&dueAmount=0.00259572
&paidCurrency=BTC
&paidAmount=0.00259572
&paidUsdt=71.594537
&paidAddress=3GJsmsMsRs4k5uGDUeRtfcWkwmovHfrtve
&confirmedTime=1685399666
&status=paid
&remark=
&unresolvedLabel=
&sign=e794933c5b0f3ff8776b299f6ba325bb72097a4b9ec9dcf32cf0a350871efb45
Asynchronous notification features
During asynchronous notification interaction, if BTPayments does not receive an HTTP response code of 200, BTPayments will consider the notification to have failed and will re-initiate notifications periodically through certain strategies. the notified interval frequencies are: 3m, 6m, 9m, 12m, 15m, 18m, 21m, 24m.
Callback notification signature verification
PHP:
public function isValid($notifyData = [], $secretKey = '') {
if (empty($notifyData) || empty($secretKey)) {
return false;
}
$str = $secretKey . $notifyData['requestId'] . $notifyData['merchantNo'] . $notifyData['orderNo'] . $notifyData['orderAmount'] . $notifyData['orderCurrency'];
$sign = hash('sha256', $str);
if ($sign === $notifyData['sign']) {
return true;
}
return false;
}
Java:
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.Map;
public class ValidationUtils {
public static boolean isValid(Map notifyData, String secretKey) {
if (notifyData.isEmpty() || secretKey.isEmpty()) {
return false;
}
String str = secretKey + notifyData.get("requestId") + notifyData.get("merchantNo") +
notifyData.get("orderNo") + notifyData.get("orderAmount") + notifyData.get("orderCurrency");
String sign = getSHA256(str);
return sign.equals(notifyData.get("sign"));
}
private static String getSHA256(String str) {
try {
MessageDigest md = MessageDigest.getInstance("SHA-256");
byte[] hashBytes = md.digest(str.getBytes());
StringBuilder hexString = new StringBuilder();
for (byte hashByte : hashBytes) {
String hex = Integer.toHexString(0xff & hashByte);
if (hex.length() == 1) {
hexString.append('0');
}
hexString.append(hex);
}
return hexString.toString();
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
return null;
}
}
}
Go:
package main
import (
"crypto/sha256"
"encoding/hex"
)
func isValid(notifyData map[string]string, secretKey string) bool {
if len(notifyData) == 0 || secretKey == "" {
return false
}
str := secretKey + notifyData["requestId"] + notifyData["merchantNo"] + notifyData["orderNo"] + notifyData["orderAmount"] + notifyData["orderCurrency"]
hash := sha256.Sum256([]byte(str))
sign := hex.EncodeToString(hash[:])
return sign == notifyData["sign"]
}
Python:
import hashlib
def is_valid(notify_data, secret_key):
if not notify_data or not secret_key:
return False
str_to_hash = secret_key + notify_data['requestId'] + notify_data['merchantNo'] + notify_data['orderNo'] + notify_data['orderAmount'] + notify_data['orderCurrency']
sign = hashlib.sha256(str_to_hash.encode()).hexdigest()
return sign == notify_data['sign']
C#:
using System;
using System.Security.Cryptography;
using System.Text;
public class Program
{
public static bool IsValid(dynamic notifyData, string secretKey)
{
if (notifyData == null || string.IsNullOrEmpty(secretKey))
{
return false;
}
string str = secretKey + notifyData.requestId + notifyData.merchantNo + notifyData.orderNo + notifyData.orderAmount + notifyData.orderCurrency;
byte[] strBytes = Encoding.UTF8.GetBytes(str);
using (SHA256 sha256 = SHA256.Create())
{
byte[] hashBytes = sha256.ComputeHash(strBytes);
string sign = BitConverter.ToString(hashBytes).Replace("-", "").ToLower();
return sign == notifyData.sign;
}
}
}
Order Status
The order status list is as follows
Order status value | Parameter description | Asynchronous notification |
---|---|---|
unpaid | Order created successfully | Yes |
pending | Payment in progress | No |
paid_confirming | User payment is successful, waiting for public chain block confirmation | Yes |
paid | The user’s payment is successful and the public chain block is confirmed | Yes |
partial_paid_confirming | The user’s partial payment is successful, pending public chain block confirmation | Yes |
partial_paid | The user’s partial payment is successful and the public chain block is confirmed | Yes |
failed | User payment failed | Yes |
Payment Currency List
The payment currency list is as follows
Currency |
---|
BTC |
ETH |
DOGE |
UNI |
XRP |
XNO |
SOL |
USDT |
USDC |
ADA |
LTC |
DASH |
SHIB |
LINK |
XLM |
ATOM |
ALGO |
DOT |
NEAR |
AVAX |
MANA |
SAND |
TRX |
APE |
ZEC |
1INCH |
AAVE |
AXS |
BAT |
BUSD |
CHZ |
COTI |
CTSI |
DGB |
EGLD |
ENJ |
EOS |
ETC |
FTM |
GAL |
GRT |
HBAR |
ICX |
ILV |
NEO |
OCEAN |
OMG |
ONT |
QTUM |
RVN |
TFUEL |
THETA |
WAVES |
XTZ |
YFI |
ZEN |
ZIL |
KAS |
Order Currency List
The order currency list is as follows
Currency | Type |
---|---|
USD | fiat |
GBP | fiat |
EUR | fiat |
HKD | fiat |
AUD | fiat |
CAD | fiat |
CHF | fiat |
BTC | fiat |
ETH | crypto |
USDT | crypto |
USDC | crypto |
Wallet list
The wallet list is as follows
Wallet ID | Wallet name |
---|---|
645 | OKX Wallet |
601 | MetaMask |
600 | Coinbase Wallet |
602 | Wallet Connect |
599 | TronLink |
SDK Lists
The SDK consists of two parts: self-built websites developed in various programming languages, third-party website-building platforms, and plugin installation packages tailored for SaaS platforms.
SDK
Development language | Version number | Last update time | Operation |
---|---|---|---|
PHP | – | – | coming soon |
NODE | – | – | coming soon |
E-commerce plugins
Platform | Version number | Last update time | Operation |
---|---|---|---|
WordPress | v0.2.1 | 2024-11-18 | Download |